Top new features of ECMAScript 2020 (ES2020)
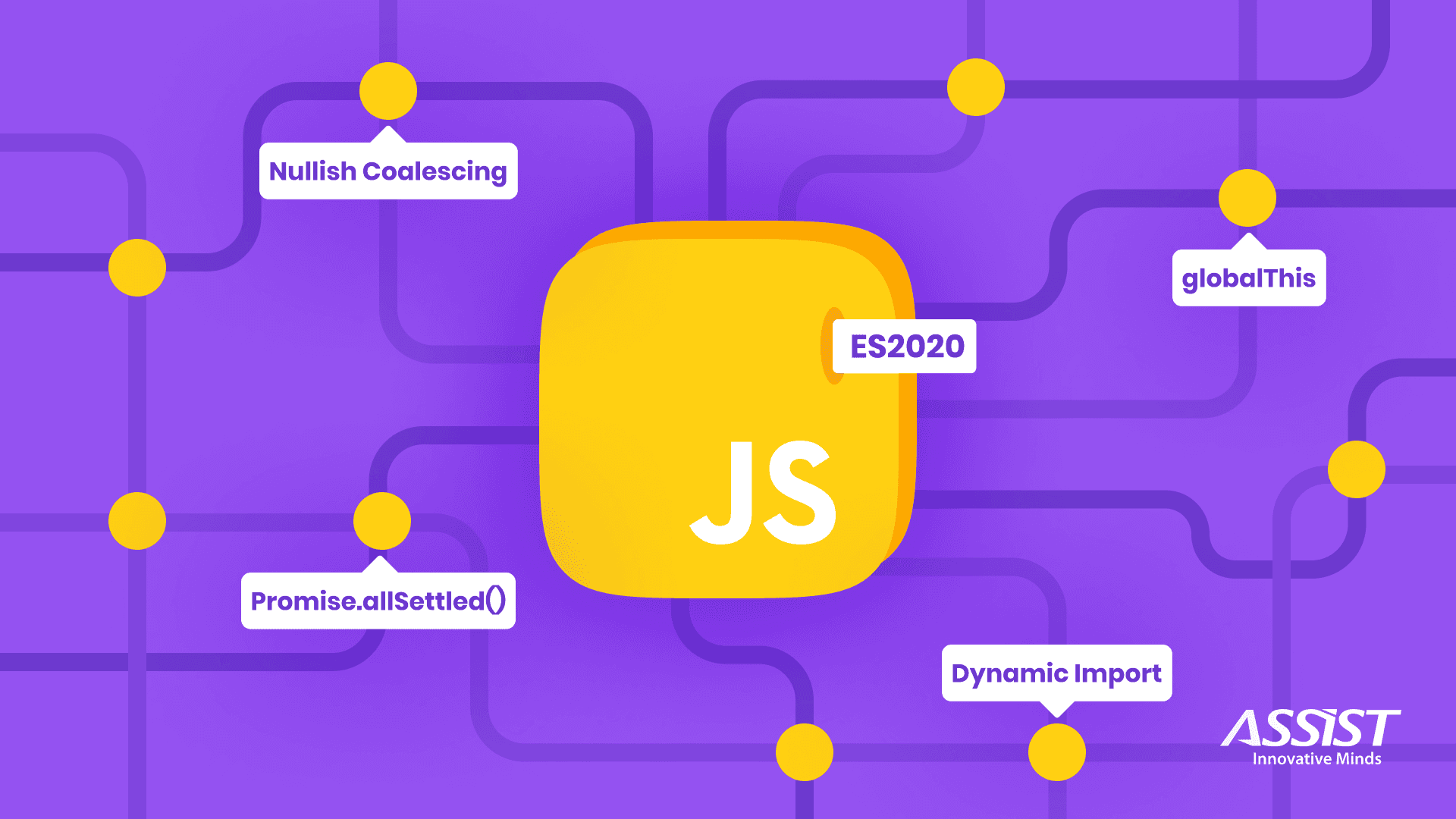
ES is short for ECMAScript, a standard that JavaScript is built on top of. Starting from 2015, the TC-39 (“a group of JavaScript developers, implementers, and more, collaborating with the community to maintain and evolve the definition of JavaScript”) (1) added new features to the JavaScript Language every year, helping us to write cleaner code and reducing as much as possible the amount of bugs in projects.
At the time of writing this article, some of the features have already been implemented. For example, Chrome 80 added support for the Nullish operator. Before using any of these new features, I recommend taking a look at https://caniuse.com/, to check if the features you want to use are available on your targeted browser.
const falsyTypes = { emptyString: '', zeroNum: 0, falseBoolean: false, nullValue: null, }
const undefinedValue = falsyTypes.undefinedValue || 'my default' console.log(undefinedValue) // 'my default' const nullValue = falsyTypes.nullValue || 'my default' console.log(nullValue) // 'my default'
const stringVal = falsyTypes.emptyString || 'my default' console.log(stringVal) // 'my default' const booleanVal = falsyTypes.falseBoolean || 'my default' console.log(booleanVal) // 'my default' const numberVal= falsyTypes.zeroNum || 'my default' console.log(numberVal) // 'my default'
const stringVal = falsyTypes.emptyString ?? 'my default' console.log(stringVal) // ' ' const booleanVal = falsyTypes.falseBoolean ?? 'my default' console.log(booleanVal) // 'false’ const numberVal= falsyTypes.zeroNum ?? 'my default' console.log(numberVal) // '0'
const nestedObject = { firstProp: { secondProp: { thirdProp: { nestedProp: 3 } } } }; // Check if the propriety exits using if statement. if ( nestedObject && nestedObject.firstProp && nestedObject.firstProp.secondProp && nestedObject.firstProp.secondProp.thirdProp && nestedObject.firstProp.secondProp.thirdProp.nestedProp ) { // do your stuff with the ‘nestedProp’. const validPropriety = nestedObject.firstProp.secondProp.thirdProp.nestedProp console.log(validPropriety) // 3 } // Check if the option exists by validating all proprieties between const validPropriety = nestedObject && nestedObject.firstProp && nestedObject.firstProp.secondProp && nestedObject.firstProp.secondProp.thirdProp && nestedObject.firstProp.secondProp.thirdProp.nestedProp console.log(validPropriety) // 3
const checkedProp= nestedObject?.firstProp?.secondProp?.thirdProp?.nestedProp console.log(checkedProp) // 5
const checkedProp = nestedObject?.inexistentProp?.secondProp?.thirdProp?.nestedProp console.log(checkedProp) // ‘undefined’
const checkedProp= nestedObject?.inexistentProp?.secondProp ?? 0 console.log(checkedProp) // 0
Let’s initialize three new Promises, two of which resolve the response and one that rejects it.
const resolvedPromise1 = new Promise(resolve => resolve("Success")); const rejectedPromise = new Promise((_,reject )=> reject("Failed")); const resolvedPromise2 = new Promise(resolve => resolve("Success"));
Promise.all([resolvedPromise1, rejectedPromise, resolvedPromise2]) .then(fianlRes => console.log("Success response: ", fianlRes)) .catch(err => console.log("Failed response:", err));

Promise.allSettled([resolvedPromise1, rejectedPromise, resolvedPromise2]) .then(fianlRes => console.log("Success response: ", fianlRes)) .catch(err => console.log("Failed response:", err));

// pdf.js export function generatePDF() { // pdf generator code }
// our document importLibrary(){ const {generatePdf} = await import('./pdf.js'); generatePDF(); } render(){ return ( <div> {/* ... */} <button onclick={()=> this.importLibrary()}>Generate pdf</button> {/* ... */} </div> ); }
// pdf.js export default function { // pdf generator code }
// our document importLibrary(){ const dinamicImport = await import('./pdf.js'); const generatePDF = dinamicImport.default; generatePDF(); }
- Web: window, self or frames;
- NodeJs: global;
- Web Workers: self.
const getGlobalObj = () => { if (typeof global !== "undefined") return global; if (typeof self !== "undefined") return self; if (typeof window !== "undefined") return window; throw new Error("Can’t get the global obj."); }; const currentGlobalObj = getGlobalObj()
const getGlobalObj = () => globalThis
class Developer { name = "Steve"; // public field #projectsInvolved = 5; // private field getNumberOfProjectsInvolved(){ return this.#projectsInvolved } getName(){ return this.name } } const dev = new Developer() console.log(dev.getName()) // "Steve" console.log(dev.name) // "Steve" console.log(dev.getNumberOfProjectsInvolved()) // 5 console.log(dev.#projectsInvolved) // Uncaught SyntaxError: Private field '#projectsInvolved' must be declared in an enclosing class
- https://tc39.es/ (1)
- proposals/finished-proposals.md at master · tc39/proposals
- Professional JavaScript for Web Developers 4th Edition - Matt Frisbie
- Nullish operator
- Dynamic Import