How to use and extend the WordPress REST API
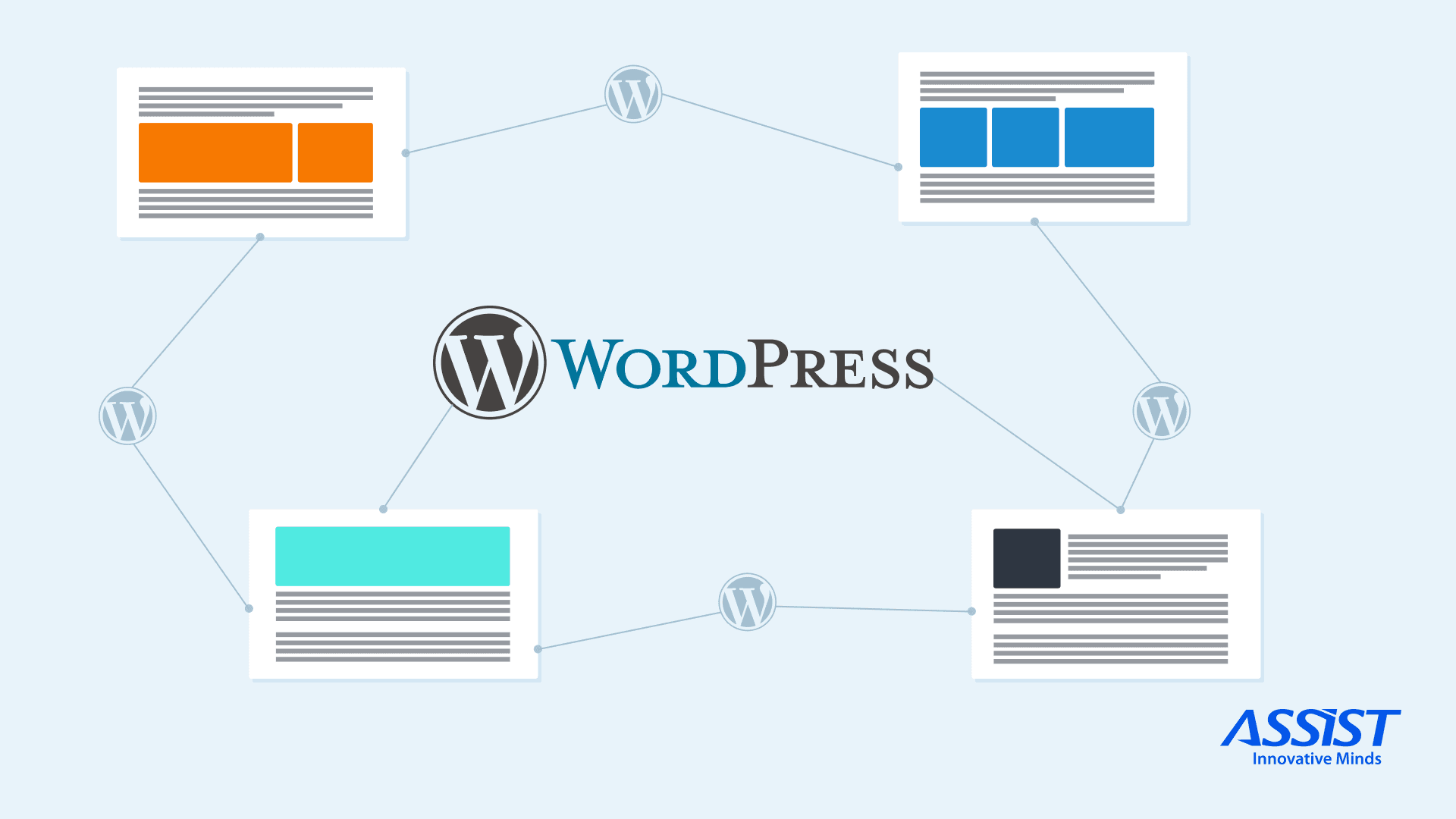
A REST API is a particular architectural approach for putting together a set of rules defined for its interaction with the wider world, which govern how other pieces of software can talk to a program and how the program will respond. REST stands for Representational State Transfer. It is a methodology designed to let programs talk to each other in as simple a way as possible.
The four main characteristics of a RESTful API are:
- Uses HTTP methods explicitly; It works over the web with standard HTTP methods such as GET, POST, PUT and DELETE.
- Is stateless; The client will include any state information needed in the request to the server and vice versa.
- Exposes directory structure-like URIs: Uses familiar URL structures like https://assist-software.net/api/v1/blogposts.
- Transfers XML, JSON or both: There is an agreed set of options for data exchange.
The WordPress REST API provides API endpoints for WordPress data types that allow developers to interact with sites remotely by sending and receiving JSON objects. JSON is an open-standard data format that is lightweight and human-readable and looks like objects do in JavaScript, hence the name.
When you send content to or make a request to the WordPress REST API, the response will be returned in JSON. This enables developers to create, read and update WordPress content from client-side JavaScript or from external applications, even those written in languages other than PHP.
Most developers think of WordPress as “that thing that’s used to create blogs”, but it is actually used in more ways than you might think. Over the past few years, WordPress has become the most popular CMS on the internet, with over 30% of the entire web built with it.
For more than three years now, the WordPress REST API has been making it easier than ever to use WordPress in new and exciting ways. For example, it has been used to create Single-Page Apps using Angular, React, and Vue. It has also been used to create Electron apps and even mobile apps using PhoneGap, Ionic or native mobile technologies on top of WordPress.
Our imagination is the only limit to what can be done with the WordPress REST API. We will talk about extending the WP API in a minute, but first, let's find out how we can start using the WP- REST API.
As long as you have a WordPress site set up, you can start experimenting with the REST API right away. You can perform various GET requests to retrieve data directly, simply by using your browser.
To access the WordPress REST API, you will need to start with the following route:
→ yoursite.com/wp-json/wp/v2
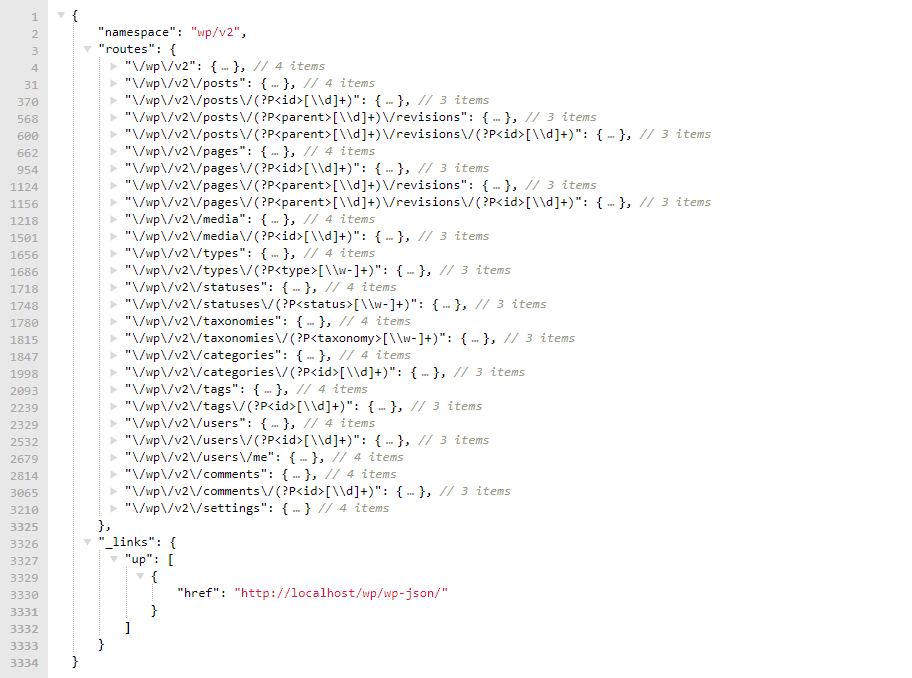
Then, you can add onto this URL to access various types of data. For instance, you could look up a specific user profile via a route like this:
→ yoursite.com/wp-json/wp/v2/users/1
In this scenario, “1” is the unique user ID for the profile you want to see. If you left out that ID, you would instead see a list of all the users on your site. Here is what I see on my local WordPress installation:
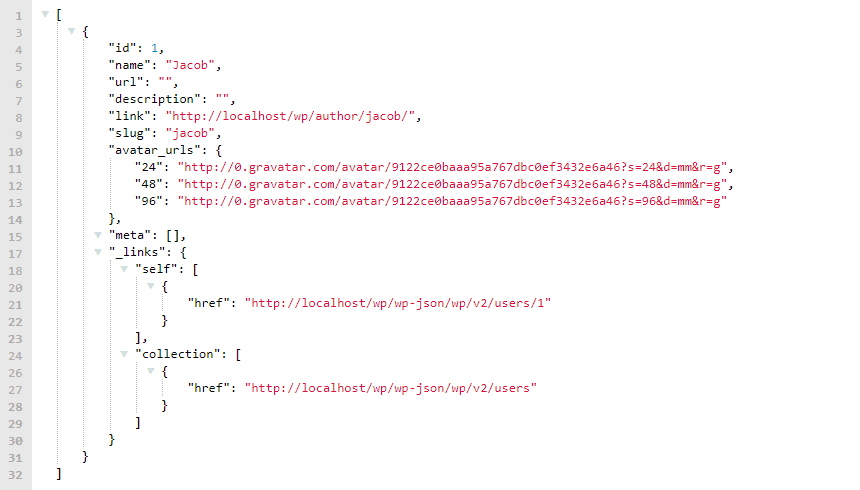
It looks like this because I use the “JSON Formatter” Chrome extension.
You can use the same basic route to view other types of data, such as your posts or pages. You can even search for subsets of the data that meet certain criteria. For example, you could retrieve all posts that include a specific term using the following URL:
→ yoursite.com/wp-json/wp/v2/posts?search=keyword
So, now that we know how we can find and use the WP-REST API, why don’t we look at an example of a real-world app?
Let’s imagine that you are a movie lover and you want to create a mobile application that shows your favorite movies. In this scenario, the WP-REST API will serve as the Back-end that will provide data to your front-end Android, iOS or PhoneGap application.
To create a new post type, you can either create a new plugin or a brand-new WP child-theme and write the custom code in its functions.php file.
In the example below, I used an existing theme and all the custom code was written in the functions.php file, as mentioned before.
/* Custom code goes below this line */ # Our custom post type function add_action('init', 'register_movie_post_type'); function register_movie_post_type() { $labels = array( 'name' => _x('Movies', 'post type general name', 'twentyseventeen'), 'singular_name' => _x('Movie', 'post type singular name', 'twentyseventeen'), 'menu_name' => _x('Movies', 'admin menu', 'twentyseventeen'), 'name_admin_bar' => _x('Movie', 'add new on admin bar', 'twentyseventeen'), 'add_new' => _x('Add New', 'movie', 'twentyseventeen'), 'add_new_item' => __('Add New Movie', 'twentyseventeen'), 'new_item' => __('New Movie', 'twentyseventeen'), 'edit_item' => __('Edit Movie', 'twentyseventeen'), 'view_item' => __('View Movie', 'twentyseventeen'), 'all_items' => __('All Movies', 'twentyseventeen'), 'search_items' => __('Search Movies', 'twentyseventeen'), 'parent_item_colon' => __('Parent Movies:', 'twentyseventeen'), 'not_found' => __('No movies found.', 'twentyseventeen'), 'not_found_in_trash' => __('No movies found in Trash.', 'twentyseventeen') ); $args = array( 'labels' => $labels, 'description' => __('This is a movie post type.', 'twentyseventeen'), 'menu_icon' => 'dashicons-editor-video', 'public' => true, 'publicly_queryable' => true, 'show_ui' => true, 'show_in_menu' => true, 'show_in_rest' => true, 'query_var' => true, 'rewrite' => array('slug' => 'movie'), 'capability_type' => 'post', 'has_archive' => true, 'hierarchical' => false, 'menu_position' => 110, 'taxonomies' => array('genre'), 'supports' => array('title', 'editor', 'author', 'thumbnail', 'custom-fields' ) ); register_post_type('movie', $args); } # Our taxonomy function add_action('init', 'create_movie_taxonomie', 0); function create_movie_taxonomie() { $labels = array( 'name' => _x('Genres', 'taxonomy general name', 'twentyseventeen'), 'singular_name' => _x('Genre', 'taxonomy singular name', 'twentyseventeen'), 'search_items' => __('Search Genres', 'twentyseventeen'), 'all_items' => __('All Genres', 'twentyseventeen'), 'parent_item' => __('Parent Genre', 'twentyseventeen'), 'parent_item_colon' => __('Parent Genre:', 'twentyseventeen'), 'edit_item' => __('Edit Genre', 'twentyseventeen'), 'update_item' => __('Update Genre', 'twentyseventeen'), 'add_new_item' => __('Add New Genre', 'twentyseventeen'), 'new_item_name' => __('New Genre Name', 'twentyseventeen'), 'menu_name' => __('Genre', 'twentyseventeen'), 'not_found' => __('No genres found.', 'twentyseventeen') ); $args = array( 'labels' => $labels, 'hierarchical' => true, 'show_ui' => true, 'show_in_rest' => true, 'show_admin_column' => true, 'query_var' => true, 'rewrite' => array('slug' => 'genre') ); register_taxonomy('genre', array('movie'), $args); }
The most important parameter in these two functions is the “show_in_rest” parameter as it lets the WP-Core know that it should include the movie post type in the WP-REST API.
Now let’s check if you successfully created the movie post type. To do this, access the same route as before: yoursite.com/wp-json/wp/v2.
You should see the following results:
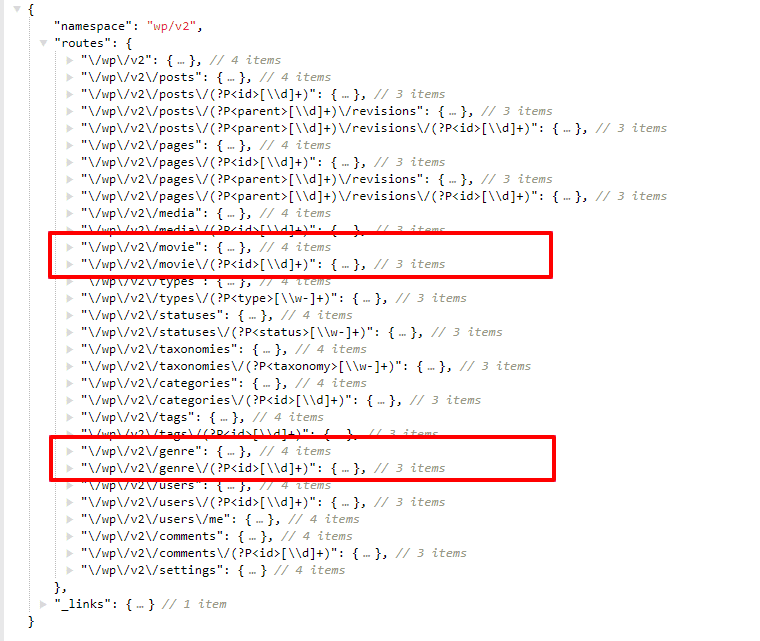
As you can see, the newly-created custom post type and its taxonomy are now showing, so you can now add new movie posts using the WP Dashboard and then fetch them through the WP-REST API.
Let me show you how this looked on my local machine after accessing:
→ http://localhost/wp/wp-json/wp/v2/movie
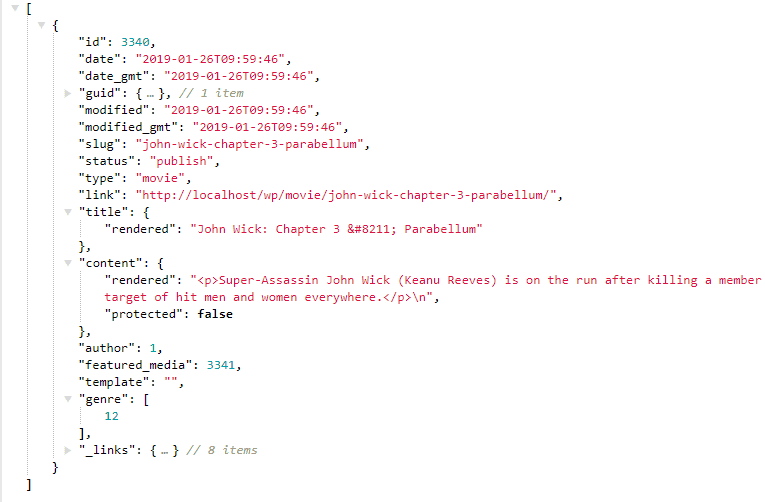
As you can see, the new movie I just added is showing as well as the ID of the genre taxonomy it belongs to.
Now that we have a movie post type registered and showing in the WP-REST API, what if you want to have something more, for example, a “rating” value?
To have this showing in the REST API, you will either have to tell the WP-Core to include the post meta to the WP-REST API too or to create a new route that accepts a post ID and returns a post metaobject, which in your case will be the movie rating value.
The first step is to register the “rating” field by accessing the “Edit Movie” screen:

Then create the new WP-REST API route to get that piece of information:
# Registering the routes in here add_action('rest_api_init', 'register_movie_routes'); function register_movie_routes() { register_rest_route('wp/v2', '/movie/rating/(?P<id>[\d]+)', array( 'methods' => 'GET', 'callback' => 'rating' )); }
Now that you have a new route under the /wp/v2/movie/ namespace, that when accessed points to the rating callback, let’s define it:
function rating(WP_REST_Request $request) { extract($request->get_params()); return [ 'rating' => get_post_meta($id, 'rating', true) ]; }
Note that I used the $id parameter in the get_post_meta function, which was made possible because I specified it when I declared the new route (?P<id>[\d]+), therefore when I used extract($request->get_params()), it automatically became available.
Let’s check if this worked by accessing it using:
→ http://yourwebsite.com/wp-json/wp/v2/movie/rating/<movie-id>
This is how it looked on my local machine:

IT WORKED!
We have seen how the WP-REST API can give us access to a very powerful backend for our mobile or web applications in a few lines of code and it is indeed only limited by our imagination.
So, if you are looking for a fast way to bring your application to life, I would recommend using the WordPress REST API.