React Basics for Beginners in 2018
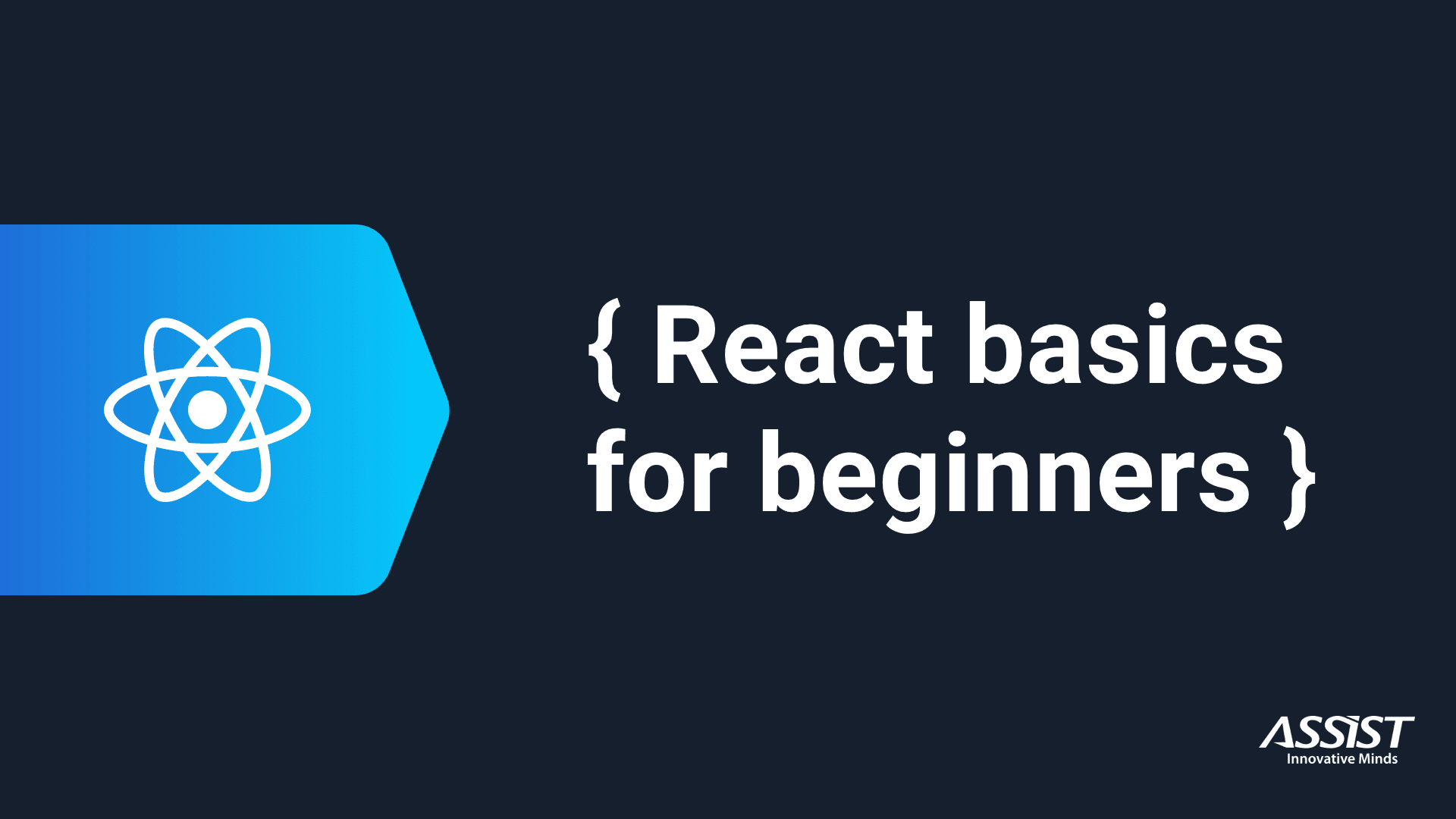
React is a JavaScript library for building interactive user interfaces. It was created by Jordan Walke, a software engineer at Facebook and is maintained now by Facebook and the community.
React isn't attempting to provide a complete 'application framework', it aims specifically for building UIs. It doesn't force one to use tons of embedded tools, but it gives the possibility to choose the preferred libraries instead. This way it allows creating a lightweight, flexible and scalable applications.
React makes it extremely simple to create interactive UIs due to its principles. It allows building encapsulated components that manage their own state and are composed to make complex UIs. React uses a "virtual DOM", which allows passing data through the app while keeping it out of the DOM. This allows the programmer to write code as if the entire page is rendered on each change, while the React libraries only render sub-components that actually change.
React can also render on the server using Node and can power mobile apps using React Native.
While experiencing many JS tools for building front-end applications, I personally found React as the simplest library for building UIs, in terms of learning and use. One can get a wide understanding of it in a very short period of time. Of course, mastering it takes time, as React is unopinionated and there often exists too much choice when implementing something. But “too much choice” becomes a strength of React when reaching a certain level of experience.
To create a new React application, one can use an integrated toolchain for the best user and developer experience. This way you can get started by skipping any configuration. The React team primarily recommends using the Create React App toolchain, as it is a comfortable environment for those who want to learn React, and is the best tool for simply building a new single-page application in React.
You’ll need to have installed on your machine a Node version greater or equal to 6 and npm version greater or equal to 5.2. To create a project named “my-app”, simply run:
npx create-react-app my-app
To start the app on the local host, run the following command from the project directory:
npm start
And that’s it, you’re ready to code. As you can see, you don’t need to install or configure tools like Webpack or Babel. They are preconfigured and hidden so that you can focus on the code.
The entry points of the app are the index.html file and are located in the public folder of the project. Inside there’s a div with a root id. This element is used to bind React.
Inside the project folder there is an src directory, containing an index.js file, which is the entry point of the scripts for the app and it binds the id of the div mentioned above with React, as follows:
ReactDOM.render(<App />, document.getElementById('root'));
This line tells the compiler that the node with the root id should be bound to the React App component. You can open the App.js file to see this default component. We will describe the components and their structure further in the next steps.
Now that we’ve passed through the setup, we’re ready to start writing an app.
JSX stands for Javascript Syntax Extension. As the name states, it is a syntax extension for JavaScript, it allows writing JS with XML-like tags, which are actually function calls that are transformed into React code and end up as HTML and Javascript in the DOM. The JSX structure of a React app is the Virtual DOM which React uses. Facebook developed an extension that helps to inspect it, called react-devtools.
Now, getting back to the App.js file and its structure. As you can see, the render method returns a JSX, and please note that it’s a mandatory thing to have everything wrapped in a single JSX node (if you don’t need to add an extra node to wrap the components, React comes with the Fragment component, which is designed to serve as a wrapper and doesn’t generate a specific DOM element after compilation).
Let’s modify this a little bit, to get a taste of it: we’re going to keep the highest-order div element and replace its content with a simple “Hello world!” text. The files should get recompiled and the browser window running the app should automatically get refreshed. Now the “Hello world!” text should be displayed in the app.
The JSX can also contain JS expressions. In order to use a JS expression in a JSX object, one should wrap the expression with curly braces. You can log to the console from JSX, call functions, make simple calculations, render elements conditionally or render lists.
{console.log('logging from JSX')} {callFunction()} {1 + 2} {isVisibile && <div>Conditionally render node</div>} <ul> {list.map(item => <li>{item.text}</li>)} </ul>
Let’s try adding an expression to our hello world message. Simply wrap the “Hello world!” text into double quotes and into curly brackets, so that the expression returned is a string. Like in the following example:
{"Hello world!"}
The app should refresh in the browser and the text should look just the same as before.
The data flow in React is unidirectional. Parent components pass data to child components. The data that a child receives from the parent is called props. To send data back to the parent, a component can use events. For example, when a certain event is triggered, like a key press or a button click, the component can handle that event by passing it to its parent, through props.
The components in React take the form of JavaScript classes. A component can be created by extending upon the React Component class. Components are used to group features, encapsulate logic and render specific JSX content. The render() method is required inside a component and it returns the JSX specific to the component, which will be compiled and rendered into HTML later. This method is triggered as a lifecycle hook when the component is being mounted and whenever the component should re-render because of an update.
Let’s create a new component to render the “Hello world” message and simply name the file "Message":
import React, { Component } from 'react'; import React, { Component } from 'react'; export default class extends Component { render() { return ( <div> {"Hello world!"} </div> ); } }
Now we can render this component as a child of our App component to display the message, by importing the newly-added component:
import Message from './Message'
and inserting it into the render method of the App component, instead of the text “Hello world!”, like this:
<Message/>
The expected state of our app is just the same as before, it should display the same message.
Lifecycle methods are hooks which allow execution of code at set points during the component's lifetime. We discussed the render method above. The main lifecycle hooks are categorized for: mounting, updating, unmounting and error handling.
At the mounting phase, the API calls are usually done inside the componentDidMount() method, while the state and data initialization are done inside the constructor().
The update lifecycle hooks are triggered whenever changes occurred to a component’s props or state. In such a case, the whole component is being re-rendered. Some update lifecycle hooks are: shouldComponentUpdate() and componentDidUpdate().
You could try adding a componentDidMount method to both our components: App and Message, and observe that the first one is executed in the App component and the second, in the Message, top-to-bottom.
As described above, the props are data that a component is getting passed by its parent. There is also one more type of data in React, called "state". The state is the private data of a component, that is locally managed and used.
The main difference between those two is that the state is private, while the props aren’t - the state can be only modified locally inside a component (as it is private), while the props can’t be changed by the component, but only by the parent component that passed them. Very often props can become a part of the state in order to be manipulated.
Let’s try passing some props from the App component to the Message component. Let’s pass the message itself as a parameter, named “text”. The syntax is:
<Message text="Hello world!"/>
And now, to display the text prop inside the Message component simply use the props instead of our expression string:
{this.props.text}
Now, we’re not able to change the text inside the Message component, but we can reuse it by displaying any text needed by any parent-component.
Handling events with React elements is very similar to handling events on DOM elements. There are some syntactic differences:
- React events are named using camelCase, rather than lowercase.
- With JSX you pass a function as the event handler, rather than a string.
Event handlers are passed instances of SyntheticEvent, a cross-browser wrapper around the browser’s native event.
Note that in JavaScript returning - false - on an event - stops the event from further propagation, while in React one should explicitly call event.preventDefault().
Let’s add an event to our app. Simply add a button to the Message component, and the click will trigger an event that we’ll propagate to the App component to update the message. Add the button to the Message component:
<button onClick={this.props.handleClick}>Update text</button>
And now, pass the click handling as a prop:
<Message text={this.state.text} handleClick={this.handleButtonClick} />
Also, don’t forget to initialize the state and define the click handling function in the App component:
state = { text: 'Hello world!' } handleButtonClick = () => { this.setState(previousState => ({text: previousState.text + ' Are you there?'})); }
Now every time the button is clicked, the event is passed to the parent, which calls the function and updates its own state and passes it to the child, which re-renders and displays the new message. It’s that simple.
Components can be classified in multiple ways. Most well-known classification would be to divide the components into Container components (that describe how things work) and Presentational Components (that are mainly about how things look).
It’s not necessary for a component to be a class, it also can be a function. So there’s also a classification of Class-based vs. Function-based components. It mostly happens that function-based components are purely presentational components that have no logic, nor state (also called stateless components) and the class-based components are usually container components, also mainly being stateful.
Here are examples of each one of them. Observe that the function-based components only render content according to the given props, they can also render other components inside, even class-based container components.
Class-based component:
import React, { Component } from 'react' class MyComponent extends Component { render() => <div>{this.props.message}</div> } Function-based component:
const myComponent = (props) => <div>{props.message}</div>
In order to add routing to your application, you may want to use the react-router-dom package. It allows taking advantage of dynamically loading routes, it exposes a Link component to use for accessing routes, the route component to declare the component that should be loaded on a specific path, the Switch component to render only one of its Routes, and many more. Routes can also be nested.
Now, you should have a basic grasp of how React works and how to start using it. As a beginner, you must know that React is simple and extremely flexible. According to the Stack Overflow survey, React grew in popularity by almost 150% from 2017 to 2018. This isn’t really surprising, and among other things, this is happening thanks to its growing component-based ecosystem. So it really worths giving it a try. I really hope you will decide diving deeper into this technology and enjoy it as much as I did.