How to Play at Work - A Play Framework Tutorial
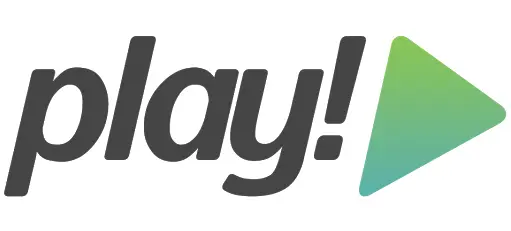
Play is an open-source modern web framework for writing scalable web applications in Java and Scala. Play is based on a lightweight, stateless, web-friendly architecture. Built on Akka, Play provides predictable and minimal resource consumption (CPU, memory, threads) for highly-scalable applications. It is developer friendly, write code, hit refresh key and see changes. Has powerful console and build tools, has great support for Eclipse and Intellij IDEA. Play was built for needs of modern web and mobile apps, provides RESTful by default, has a built-in JSON module and extensive NoSQL & Big Data Support. It is solid, fast and the code is compiled and runs on JVM. The ecosystem around Java is huge. There are libraries for everything - most of which can be used in Play. "Play Framework is the best".
Below are the minimum system specifications to develop an application in Play Framework for Windows, Mac and Linux.
Note: A list with more tools to play with you find here.
- Install JDK 1.6 or later.
- If you are using MacOS, Java is built-in.
- If you are using Linux, make sure you use either the Sun JDK or OpenJDK.
- If you are using Windows, just download and install the latest JDK package.
- Install Scala is a general-purpose language designed to support clear, concise and type-safe programming as well as to scale from the smallest applications to the biggest. (Optional, but good to have, you may also use Java).
- Install SBT is an open source build tool for Scala and Java projects, similar to Java's Maven or Ant. (Optional, but good to have).
- Install Play Framework through a tool called Typesafe Activator.
- Install PostgreSQL.
- Install pgAdmin is a comprehensive PostgreSQL database design and management system (Windows and Mac, pgAdmin is included by default in most Linux distributions, such as RedHat, Fedora, Debian and Ubuntu).
Here is a Github project source where you can find all the work that will be explained below and some aditional examples.
Let's create a new vanilla Play Scala application and name it scala-game. Here is the command you need to run it in command-line:
activator new scala-game play-scala
To start your game from command-line run from your project root folder:
activator run
or (recommanded)
sbt run
To view your work go to: localhost:9000/
Refresh the page every time you made changes and want to see them.
To add more experience to your Play skills add the following dependencies to your build.sbt file:
"com.typesafe.play" %% "play-slick" % "0.8.1", "org.postgresql" % "postgresql" % "9.2-1002-jdbc4"
- Slick is a modern database query and access library for Scala. It allows you to work with stored data almost as if you were using Scala collections while at the same time giving you full control over when a database access happens and over which data is transferred. You can write your database queries in Scala instead of SQL, thus profiting from the static checking, compile-time safety and compositionality of Scala.
- PostgreSQL JDBC is necessary to connect to PostgreSQL database
Now, if you have this dependencies, create a database and add its credentials to application.config file. Add a package named dao to your app folder, where we define database tables and set it in configuration file to tell Slick where the table mappings are, also uncomment evolution plugin and set it enabled so that Play Framework's Evolutions will create tables and relations between them (primary keys, indexes, sequences etc...) for you.
db.default.driver=org.postgresql.Driver db.default.url="jdbc:postgresql://localhost:5432/scalagame" db.default.user=postgres db.default.password="password" # Evolutions # ~~~~~ # You can disable evolutions if needed slick.default="dao.*" evolutionplugin=enabled
To make the application to suit our needs we have to specify to which database to connect (a Play application can define muliple databases, we have just one named default, but we can define other databases as well ex: db.history where we can store player's history, etc ...), to do that we need to create a trait named PostgresSupport and define an implicit database session so won't need to set it out for every request to database:
import play.api.Play.current import play.api.db.slick.Config.driver.simple._ trait PostgresSupport { trait PostgresSupport { implicit lazy val session: Session = play.api.db.slick.DB("default").createSession() } }
Create a package models and in it a case class named Player. Complete models
case class Player ( idPlayer: Option[Int], name: String, level: Int )
Having our modeles we just need to map them to tabels. As you see in the model idPlayer is optional because we will let PostgreSQL to generate a PrimaryKey for the player using AutoInc. Complete tables
class PlayerTable(tag: Tag) extends Table[Player](tag, "players") { def idPlayer = column[Int]("id_player", O.PrimaryKey, O.AutoInc) def name = column[String]("name", O.NotNull) def level = column[Int]("level", O.NotNull) def * = (idPlayer.?, name, level) <> (Player.tupled, Player.unapply _) }
Play tracks your database evolutions using several evolution scripts. These scripts are written in plain old SQL and should be located in the db/evolutions directory of your application. The first script is named 1.sql.
If evolutions are activated, Play will check your database schema state before each request in DEV mode, or before starting the application in PROD mode. In DEV mode, if your database schema is not up to date, an error page will suggest that you to synchronize your database schema by running the appropriate SQL script.
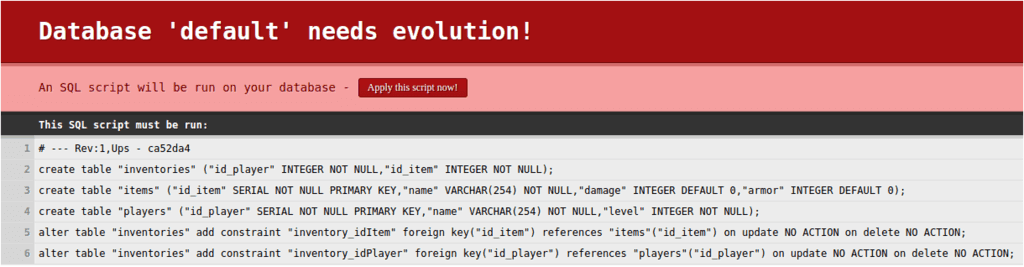
When a user first time accesses our application on localhost "localhost/" a GET HTTP request will be made to this route "/". The entire list of routes can be found in the conf/routes file, it is the configuration file used by the router. This file lists all of the routes needed by the application. Each route consists of an HTTP method and URI pattern, both associated with a call to an Action generator.
# Home page GET / controllers.Application.index # Insert single player POST /player/single controllers.Application.addSinglePlayer # Get Players Json Format GET /player/multi controllers.Application.findAll # Insert multi player Json format POST /player/multi controllers.Application.addMultiPlayer # Map static resources from the /public folder to the /assets URL path GET /assets/*file controllers.Assets.at(path="/public", file)
Most of the requests received by a Play application are handled by an Action. An Action is basically a request => result function that handles a request and generates a result to be sent to the client. As you can see every route in our routes file is accessing a method of an object Application aka Controller in controllers package. A Controller is nothing more than a singleton object that generates Action values.
You can chose to Play in two ways: Single player using Play Scala template or Multi player using one of the follwing JavaScript frameworks: AngularJS, BackboneJS, EmberJS, ExtJS, DustJS or other JavaScript frameworks used to develop web applications.
Adding a new player and get players list using Slick.
- players list (players is the mapped table)
def findAll = players.list
- add new player
def savePlayer(player: Player): Player = { player.idPlayer match { case None => { val id = (players returning players.map(_.idPlayer)) += player player.copy(idPlayer = Some(id)) } case Some(id) => { val query = for { c <- players if c.idPlayer === id } yield c query.update(player) player } } }
A Play Scala template is a simple text file, that contains small blocks of Scala code. They can generate any text-based format, such as HTML, XML or CSV. The template system has been designed to feel comfortable with those used to dealing with HTML, allowing web designers to easily work with the templates. Templates are compiled as standard Scala functions.
If you create a views/Application/index.scala.html template file, it will generate a views.html.Application.indexfunction. By accessing / route with a GET request will be sent to the server, the router will call controllers.Application.index which will return as a response a complete list of players (HTML format).
object Application extends Controller { def index = Action { Ok(views.html.index(Players.findAll)) } }
In index.scala.html we have the player list, so we just need to iterate it and display every player in a table. Play Framework will render the page server-side.
@(players: List[Player]) @main("Player List") { <h2>Previously inserted players:</h2> <table> <tr><th>idPlayer</th><th>Name</th><th>Level</th></tr> @for(p <- players){ <tr><td>@p.idPlayer</td><td>@p.name</td><td>@p.level</td></tr> } </table> }
Adding players to the game is as simple as that: submit a form using POST to this route /player/single
<h2>Insert a player here:</h2>
<form action="/player/single" method="POST">
<input name="name" type="text" placeholder="player name"/>
<input name="level" type="text" placeholder="player level"/>
<input type="submit"/>
</form>
will call this method controllers.Application.addSinglePlayer.
def addSinglePlayer = DBAction { implicit rs => val player = playerForm.bindFromRequest.get Players.savePlayer(player) Redirect(routes.Application.index) }
Multi Player
Building a modern web application, with a client-side JavaScript application, served from the Play Framework, they will be two separeted applications in one project: API Backend (presistent data) and Frontend side (making AJAX calls to the server). The JavaScript application will be in public folder, aiming to server static content: HTML, CSS, images, etc ...
To test your calls use Postman.
By making a GET call to /player/multi this method will execute controllers.Application.findAll and it will return a list of players in JSON format.
def findAll = DBAction{ implicit rs => Ok(Json.toJson(Players.findAll)) }
Example:
[ { "idPlayer": 1, "name": "Frunza", "level": 21 }, { "idPlayer": 2, "name": "Gamer", "level": 41 } ]
To add a player use POST method to this route /player/multi with the following JSON body:
{ "name": "Newbie", "level": 1 }
Game Features
To gain more experience in Playing at work you can try this modules or create one by yourself and support the community.
- Amazon S3 module (Scala). A minimal S3 API wrapper that allows you to list, get, add and remove items from a S3 bucket.
- Authentication and Authorization module (Scala). This module offers Authentication and Authorization features to Play2.x applications.
- Deadbolt 2 Plugin. Deadbolt is a powerful authorisation mechanism for defining access rights to certain controller methods or parts of a view.
- Dust Plugin. Provides support for the dust client side template language (DustJS).
- Memcached Plugin. Provides a memcached based cache implementation.
- MongoDB Salat, Casbah Plugin (Scala). Provides managed MongoDB access and object mapping using Salat and Casbah
- Redis Plugin (Java and Scala). Provides a redis based cache implementation, also lets you use Redis specific APIs
- SecureSocial (Java and Scala). An authentication module supporting OAuth, OAuth2, OpenID, Username/Password and custom authentication schemes.
Most popular Players
- The Guardian
- Foursquare
- Coursera
- Klout
- Walmart
Hope you guys get some uses out of this Play Framework tutorial, and start developing awesome web applications for your own.